GUI Programming In Python
GUI stands for Graphical User Interface. GUI is commonly used interface to interact with the Application.
GUI application is very simple to use and more effective than command based Application.
GUI Programming in Python
Python programming language is also used to create GUI Application and Python has a GUI library, which is called Tkinter.
In Python, Windows form can be developed in few lines of code.
In Python programming language, we can develop GUI Application more easily than other programming language.
Python GUI Tools Kits
Python provides many GUI tool kits to develop GUI Application but most common are listed below-
Python GUI Tools Kits
Python provides many GUI tool kits to develop GUI Application but most common are listed below-
- Tkinter: Tkinter is Python GUI programming tool kit and Tkinter is most commonly used.
- wxPython: This is an open-source Python interface for wxWindows, which can be downloaded from http://wxpython.org.
- JPython- JPython is a Python port, which provides an access of Java library and also provides importing facilities. You can download from http://www.jython.org.
Tkinter is a powerful Python GUI development library and Tkinter is an object oriented programming tool kit.
Tkinter provides the faster GUI development environment with Tk tool kit.
Creating GUI Application
In Python programming language, GUI development is very easy, as following steps needs to be followed-
- Importing main Tkinter module.
- Creating main Window.
- Add Tkinter widgets into main Window.
- Enter the main loop to take an action against the event, which is triggered by the user.
- import tkinter
- main=tkinter.Tk()
- main.mainloop()
Output-
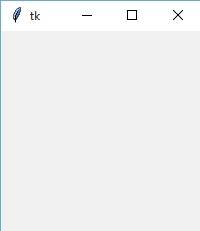
Tkinter Widgets
Tkinter provides the various control to add more functionality into our GUI Application.
Most common Tkinter widgets are listed below-
Widgets | Description |
Button | The Button widget is used to add buttons in our Application. |
Canvas | The Canvas widget is used to draw shapes, such as lines, ovals, polygons and rectangles in our Application. |
Checkbutton | The Checkbutton widget is used to display a number of options as checkboxes. The user can select multiple options at a time. |
Entry | The Entry widget is used to display a single-line text field to accept the values from a user. |
Frame | The Frame widget is used as a container widget to organize other widgets. |
Label | The Label widget is used to provide a single-line caption for other widgets. It can also contain the images. |
Listbox | The Listbox widget is used to provide a list of options to a user. |
Menubutton | The Menubutton widget is used to display menus in your Application. |
Menu | The Menu widget is used to provide various commands to a user and user selects to perform an operation. |
Message | The Message widget is used to display multiline text fields to accept the values from a user. |
Radiobutton | The Radiobutton widget is used to display a number of options as radio buttons. The user can select only one option at a time. |
Scale | The Scale widget is used to provide a slider widget. |
Scrollbar | The Scrollbar widget is used to add scrolling capability widgets, such as list box etc. |
ext | The Text widget is used to display the text in the multiple lines. |
Toplevel | The Toplevel widget is used to provide a separate Window container. |
Spinbox | The Spinbox widget is a variant of the standard Tkinter Entry widget, which can be used to select from a fixed number of values. |
PanedWindow | A PanedWindow is a container widget, which may contain any number of panes, arranged horizontally or vertically. |
LabelFrame | A labelframe is a simple container widget. Its primary purpose is to act as a spacer or a container for complex Window layouts. |
tkMessageBox | This module is used to display message boxes in your Applications. |
Summary
In this chapter, you learnt the basics of GUI programming, Tkinter module of Python programming, how to create parent Window in Python, Tkinter Widgets.