Tkinter Widget-Entry
Entry is used to accept the strings from a user.
Syntax-
w = Entry( main, option, ... )
Main- Parent Window.
Option- attribute of button widget.
Example-
- from tkinter import *
- main= Tk()
- L1 = Label(main, text="User Name")
- L1.pack( side = LEFT)
- E1 = Entry(main, bd =5)
- E1.pack(side = RIGHT)
- main.mainloop()
Output-
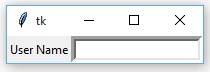
Option | Description |
bg | Normal background color. |
bd | Sets the border width in pixels. Default is 2. |
command | It is used to call a method or a function. |
cursor | Cursor is used to create a cursor in canvas like arrow, circle, dot etc. |
font | It is used to set the font style. |
exportselection | It is used to avoid the selection of the text on the clipboard. |
fg | It sets the normal foreground color of the text. |
highlightcolor | Changes color of widgets on focus. |
justify | It is used to justify the text of widgets. |
relief | Relief is special type of border. The values are SUNKEN, RAISED, GROOVE and RIDGE. |
selectbackground | It is used to set the background color. |
selectborderwidth | It is used to set the border width around the selected text. Default 1 px |
selectforeground | It is used to set foreground color of the selected text. |
show | It is used to set the type of text is appearing in entry like simple text or password. |
state | It is used to set the state of widgets, where the state is ACTIVE or DISABLE. |
textvariable | It is used to store a value of an entry. |
width | It is used to set the width of widgets. |
xscrollcommand | It is used to scroll on the defined value in x direction |
Summary
In this chapter, you learnt what Entry widget is and how to use the property of the widget.