Loops In Python
Introduction
In this article, you will learn that programming language statement is executing sequentially, which means first statement is executing first then second so on.
If we want to execute one statement more than one time, loops provide a facility to execute the statement according to our condition.
In other words, an iteration of single statement or a block of statement can be used for the looping statement in Python.
Flow chart
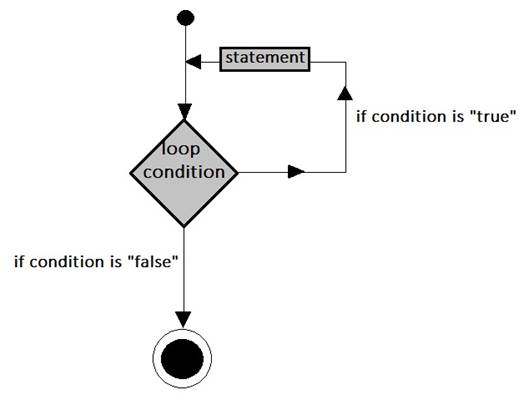
Python supports following loop statement
- while loop
- for loop
- nested loop
While loop
While loop is used for an iteration of the statement according to the condition. While loop checks condition first, if condition is “true”, it executes the statement.
Flow chart
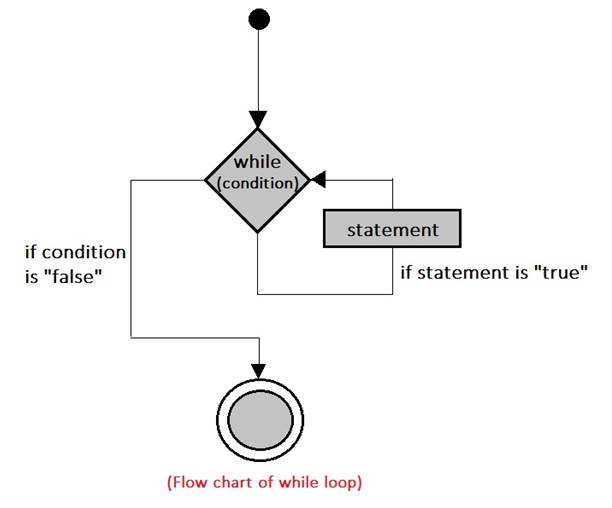
Syntax
while condition:
statement(s)
key note statement(s) can be individual or group of statement.
Example
- n = int(input("enter a number"))
- i = 1
- while i <= 10:
- print(n, "X", i, "=", i * n)
- i = i + 1
Output
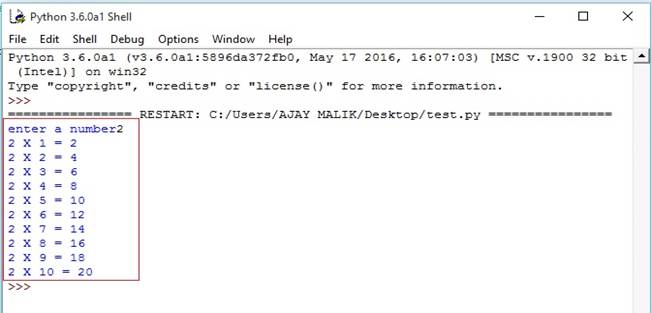
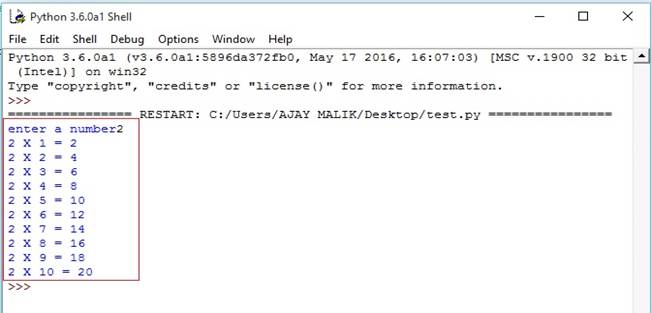
Explanation
In the example, shown above, a user enters a number and prints a table of the number. While loops execute 10 times and prints the table of the number.
for loop
“for loop” has an ability to iterate the item of sequence. For example, list or string etc. “for loop” iterates an item in a sequence.
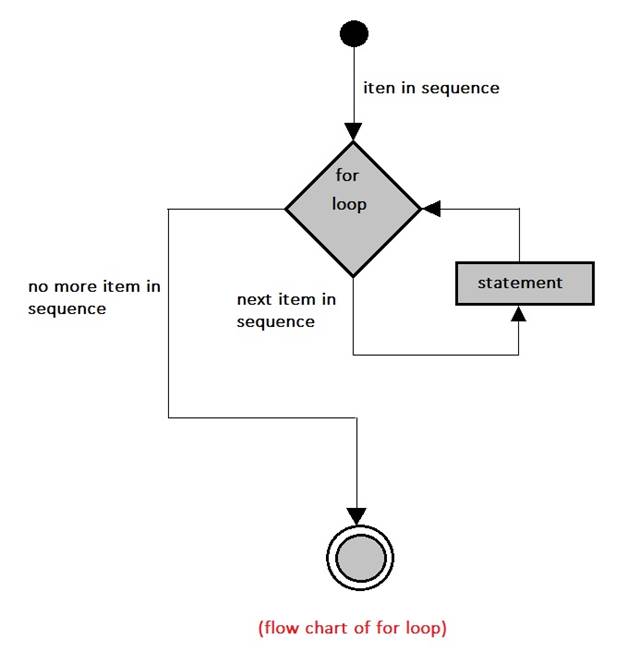
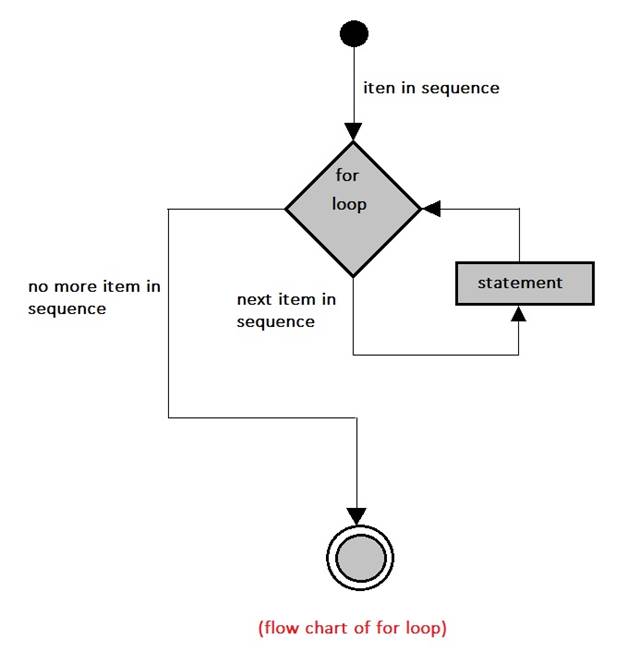
Syntax
for iteration_variable in sequence:
statements(s)
Example
- tech=[".net","asp.net","c#.net","mvc"] #list of technology
- for item in tech: #for loop "item is iteration variable" and "tech is list
- print("technology name: ",item)
- print("end of list")
Output
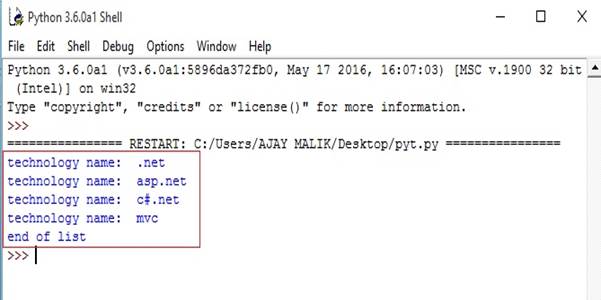
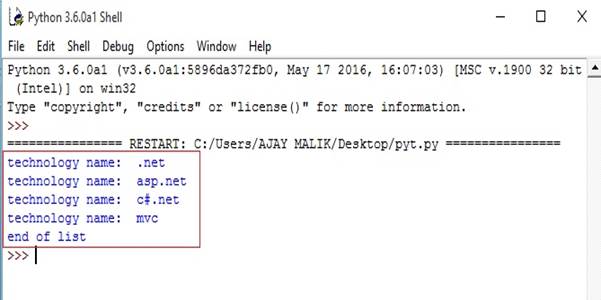
Explanation
For loop iterates every item from the list.
Example
Print the table for a number, using for loop.
- n = int(input("enter a number"))
- for i in range(1, 11):
- print(n, "x", i, "=", n * i)
Output
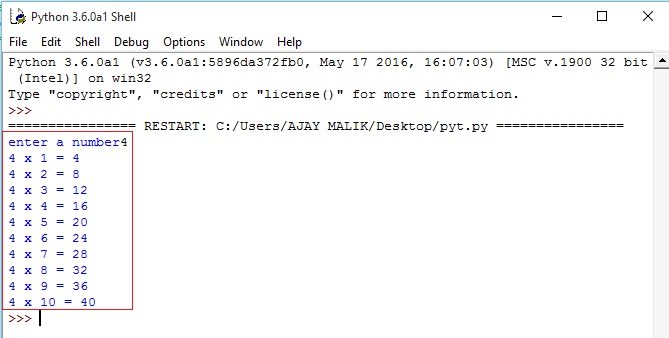
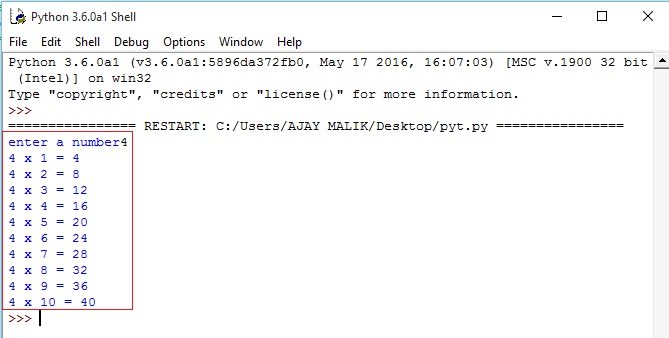
Explanation
In this program, a user enters a number to print the table of an input number and ‘for loop’ iterates ten times according to range() function and finally prints table of the number.
Nested loop
In Python programming language, nested loop is one loop inside another loop.
We put any type of loop inside any other type of loop in Python for example we put “for loop” inside “while loop” or vice versa.
We put any type of loop inside any other type of loop in Python for example we put “for loop” inside “while loop” or vice versa.
Generally, it is used for pattern print
Syntax
“while loop” inside “while loop”
while condition:
while condition:
statement(s)
Example
“while loop” inside “while loop”
- str = ''
- i = 1
- while i <= 5:
- j = 1
- while j <= i:
- str = str + "*"
- j = j + 1
- i = i + 1
- str = str + "\n"
- print(str)
Output
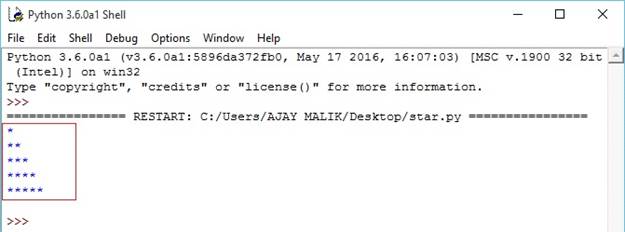
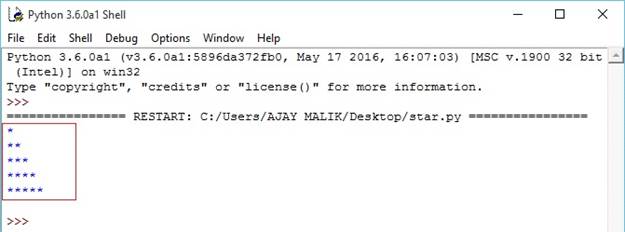
Explanation
In this program outer ‘while loop’ iterates five times and inner ‘while loop’ iterates according to the condition outer ‘while loop’ and ‘*’ is add to ‘str’ in inner ‘while loop’ according to iteration and ‘\n’ is also added in every iteration of outer ‘while loop’.
Syntax
“for loop” inside “while loop”
While condition:
for iteration_variable in sequence
statement(s)
Example
“for loop” inside “while loop”
- str = ''
- i = 1
- while i <= 5:
- for j in range(0, i):
- str = str + "*"
- i = i + 1
- str = str + "\n"
- print(str)
Output
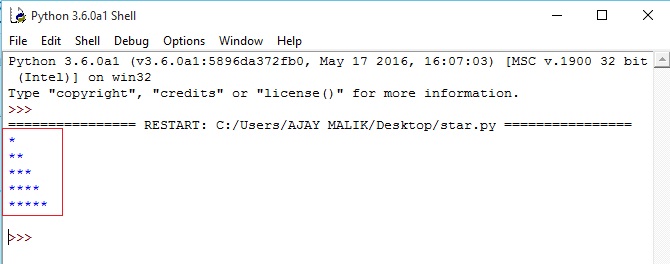
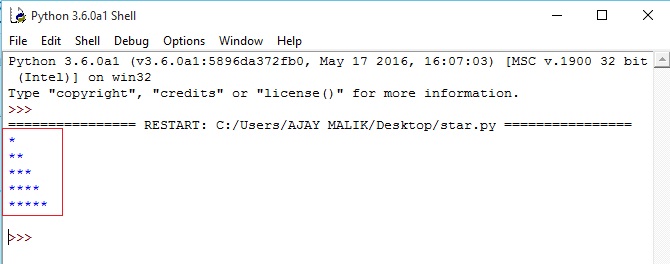
Explanation
In this program, ‘while loop’ iterates five times and ‘for loop’ iterates according to the condition of ‘while loop’ and ‘*’ is added to ‘str’ in ‘for loop’, according to iteration and ‘\n’ is also added in every iteration of ‘while loop’.
Syntax
“for loop” inside “for loop”
“for loop” inside “for loop”
for iteration_variable in sequence:
for iteration_variable in sequence:
statement(s)
Example
“for loop” inside “for loop”
- str = ""
- for i in range(1, 6):
- for j in range(0, i):
- str = str + "*"
- str = str + "\n"
- print(str)
Output
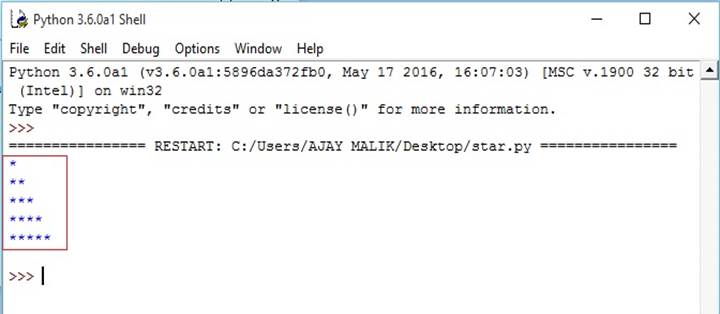
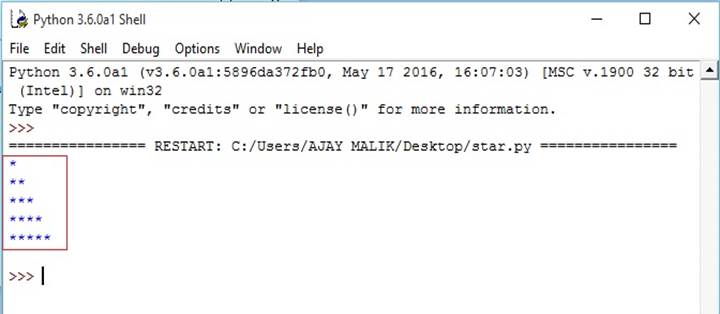
Explanation
In this program ‘for loop’ iterates five times and inner ‘for loop’ iterates according to iteration variable of outer ‘for loop’ and ‘*’ is added to ‘str’ in inner ‘for loop’, according to iteration and ‘\n’ is also added in every iteration of outer ‘for loop’.
Syntax
“while loop” inside “for loop”
for iteration_variable in sequence:
while condition:
statement(s)
Example
“while loop” inside “for loop”
- str = ''
- for i in range(1, 6):
- j = 0
- while (j < i):
- str = str + "*"
- j = j + 1
- str = str + "\n"
- print(str)
Output
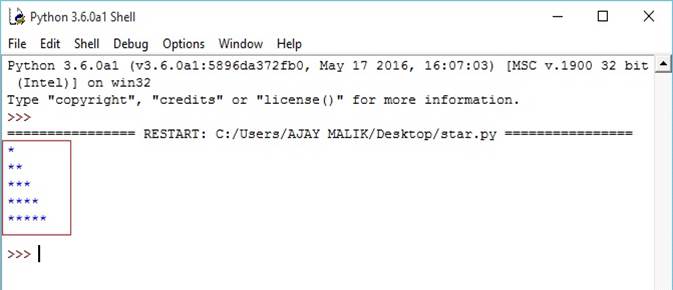
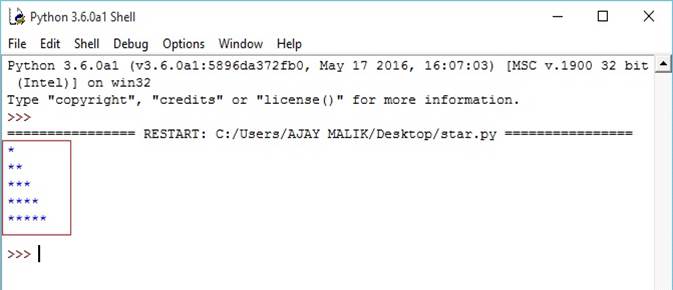
Explanation
In this program, ‘for loop’ iterates five times and ‘while loop’ iterates according to iteration variable of ‘for loop’ and ‘*’ is added to ‘str’ in ‘while loop’ , according to iteration and ‘\n’ is also added in every iteration of ‘for loop’.
Loop control statement
In Python, control statement is used to change the execution of the loop for its normal sequence.
Python supports the following control statements.
- break statement
- continue statement
- pass statement
Break statement is used to terminate the loop on the certain condition and next statement is executed as well, which means it only terminates the block of loop and does not effect on any other statement outside the block of the loop.
Break can be used with “for loop” as well as “while loop”.
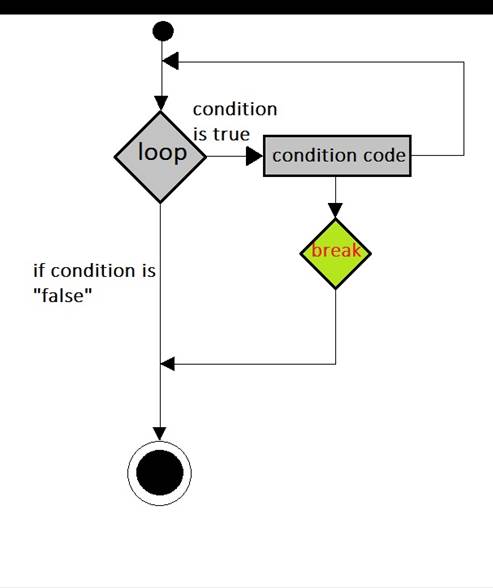
Syntax
break #We only write break for the termination of the loop and the block of the loop.
Example
- list = ['.net', 'C#', 'asp.net', 'mvc']
- name = input("enter a technology for search")
- for item in list:
- if item == name:
- print("name is found")
- break
- else :
- print(name, "is not found.. wait check in next item")
Output
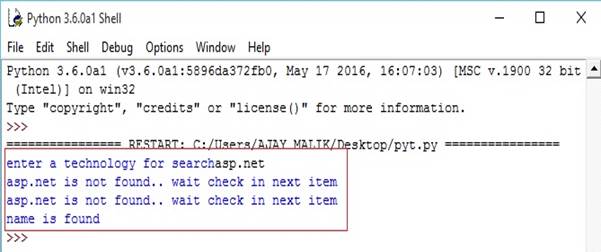
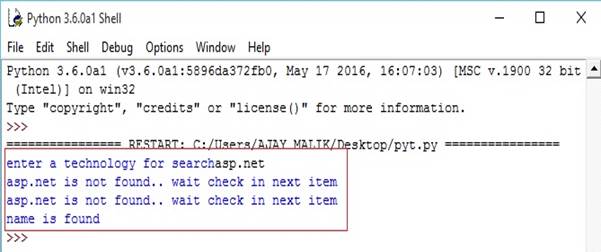
Explanation
In the program, mention the user, enter a technology and search in the list, “for loop” iterates all the item of the list and technology is found then “for loop” is terminate.
Continue statement
Continue statement is used to skip statement inside the loop block and continue statement returns control of the loop and moves back to beginning.
Control statement is used with “for loop” and also while loop.
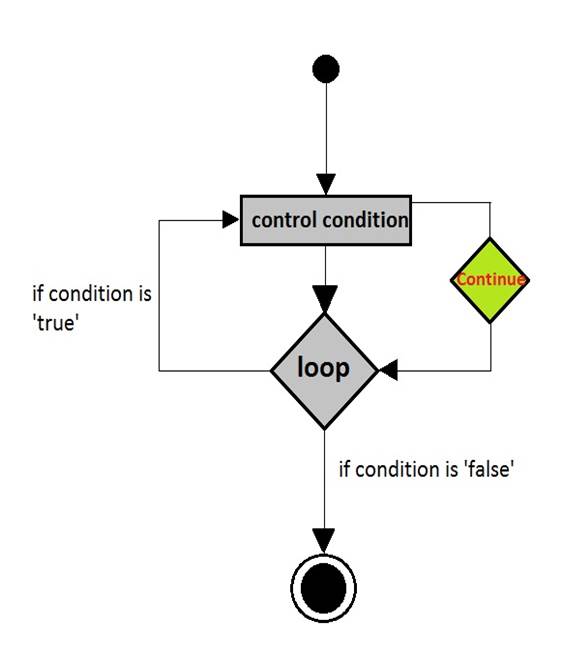
Syntax
Control statement is used with “for loop” and also while loop.
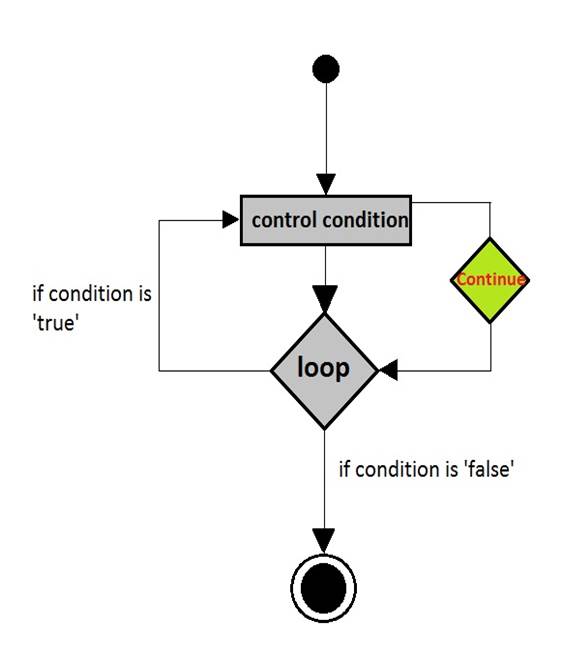
Syntax
Continue # only write continue.
Example
- n = int(input("enter a range"))
- i = 0
- print("\neven number are in range 1 to", n)
- while i <= n:
- i = i + 1
- if i % 2 != 0:
- continue
- print(i)
Output
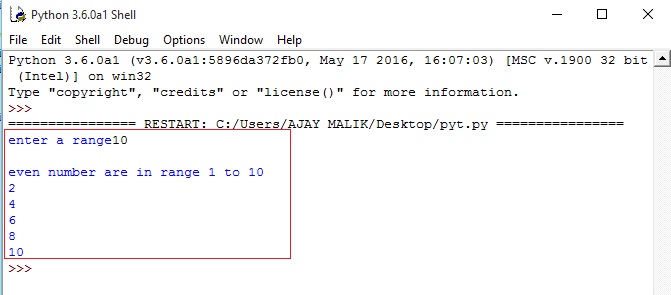
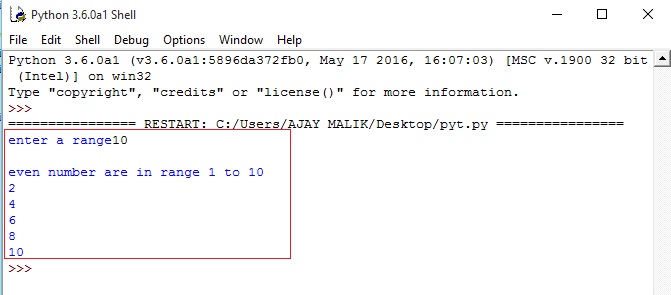
Explanation
In the program, shown above, the user enters a range and while loop iterates the loop according to the range and “if condition” checks the number, which is odd, if this condition is skipped, using continue statement and prints only even number.
Pass statement
Pass statement is null operation, pass statement does nothing on execution but it is used as a placeholder in our program, when we need a statement syntactically.
Syntax
pass #only write pass
Example
- for i in 'c#corner':
- if i=='#':
- pass
- print("charater=",i)
Output
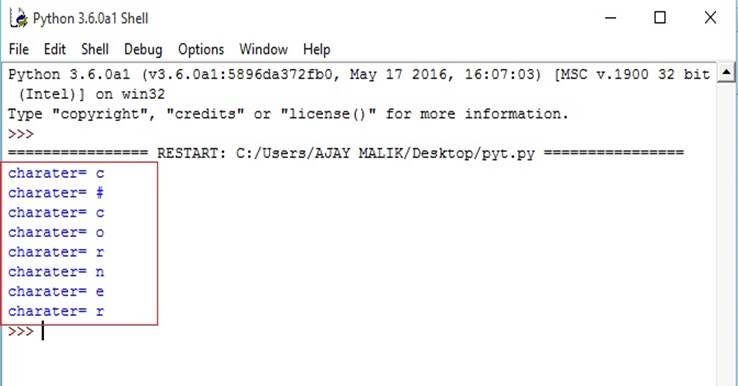
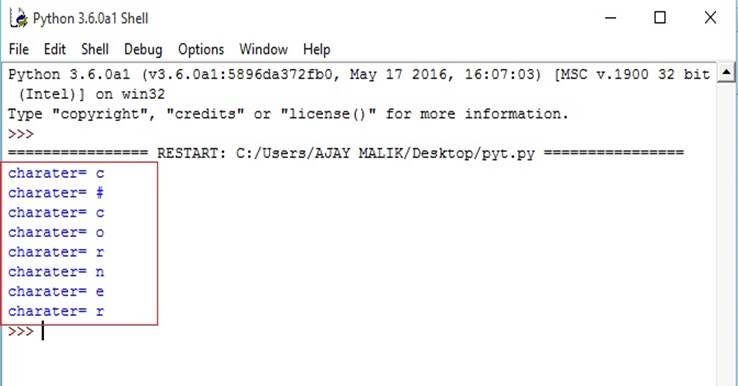
Explanation
In the program, mentioned above, we put pass statement in “if condition” but if condition does not affect the sequence and the sequence is printed exactly same.
Summary
In this article, you learnt the concept of looping, types of loops like while loop and for loop etc.